1. Define such a, that expression would resolve to true
(a == 0) && (a == 1) && (a == 2)
var a = {
i: 0,
valueOf: function() {
return this.i++;
}
};
(function(global) {
var value = 0;
Object.defineProperty(global, 'a', {
get: function() {
return value++;
}
});
})(window)
a
in Method 1 is Object's type instead of Integer's type of Method 2. It leads above expression is only equal in term of value.a
in Method 2 can not be changed or listed differ to in Method 1.- There's no way to modify value of
a
in Method 2.
- Numberic
toString
: happens when a string representation of an object is required. - String
valueOf
: performs two cases- In function which need a number, for example
Math.sin(obj)
,isNaN(obj)
or+obj
. - In comparison like
==
but not for===
- In function which need a number, for example
- Boolean.
2. What's the different between EMACScript and Javascript?
- EMACScript is an object-oriented programming language for performing computations and manipulating objects within a host environment.
- Javascript is one of well-known EMACScript's dialect.
3. Why does nearly every object have a toString method?
toString
will be called automatically.The default
toString
function will return [object type]
.Common way to use is to detect object class
var toString = Object.prototype.toString;
toString.call(new String) // [object String]
toString.call(undefined) // [object Undefined]
4. Do you know which interpreter Mozilla Firefox uses? Or any other of the browsers?
- Mozilla Firefox: SpiderMonkey
- Chrome: V8
- Safari: Nitro
- Internet Explorer: Chakra
5. Is there block scope in JavaScript?
For that reason, it is recommended to always declare variables at the top of their scope (the top of global code or function code) and declares functions at the bottom of their one.
6. Can you explain how Ajax/XMLHttpRequest works?
This picture below will describe how it works
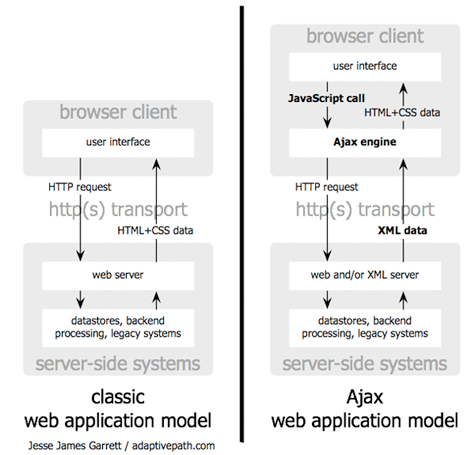
The different between ajax and classic web
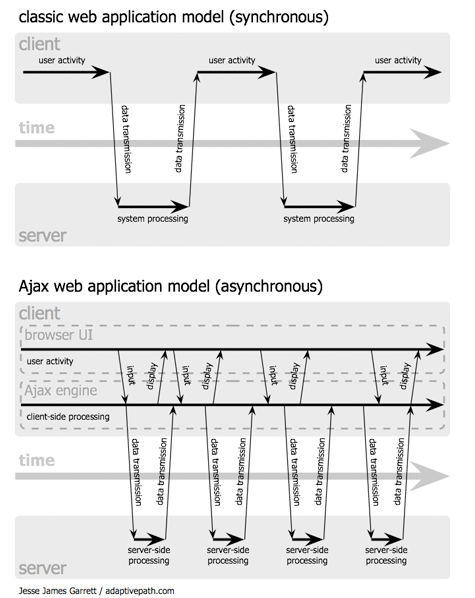
7. What is JSON?
JSON object contains methods for parsing Javascript Object Notation and converting values to JSON. It can't be called or constructed and aside from its method properties(
JSON.parse()
and JSON.stringify()
) it has no interesting functionality of its own.✍ JSON is not subset of javascript. In general, JSON spec says that "a string is a sequence of zero or more Unicode characters, wrapped in double quotes, using backslash escapes." But in javascript "a string can contain anything as long as it's not a quote, a backslash or line terminator(\u000A, \u000D, \u2028, \u2029)", therefore two invisible Unicode characters(U+2028, U+2029) are the resason.
For example:
{"JSON":"ro
cks!"}
8. An example of a singleton pattern?
(function(global) {
var instance;
function Singleton() {
if (!instance) {
instance = this;
} else {
return instance;
}
};
global.Singleton = Singleton;
})(this);
new Singleton() === new Singleton()
9. What's the difference between undefined
and undeclared
?
undefined
is the one of built-in types. it has a mean that the variable was declared but not initialized. There are three ways to achive 'undefined':
- declare variable
- access not existing property of object
undeclared
means that the variable wasn't declared in chaining scope.declared vs undeclared variables also have mean like that
var declared = 1 // explicit
undeclared = 1
10. Are you familiar with Web Workers
?
11 The standard addEventListener
is supported in which browsers?
addEventListener
is a method registers the specified listener on EvenTarget. The even target may be an Element in document, Document itself, a Window or any other object that supports events(such as XMLHttpRequest).It is supported in most of browsers except IE 9.
When talking about
addEventListener
, people often compare it with inline events. Inline events are better in case of browser compatibility but it has many drawbacks.
- You may only have one inline event assigned.
- The context
this
in case of inline event is global compare to HTML element in case ofaddEventListener
.
addEventListener
but has some important callbacks.
- Events always bubble, no capturing possibility.
this
always refers to the windows.
12. What is the difference between ==
and ===
? Which one would you use?
==
compares only value while ===
compares value and type.The operand
==
leads to type conversion, when type conversion is involved in comparsion(ie. non-strict mode) Javascript converts String
, Number
, Boolean
or Object
operands as follows:
Number
vsString
: try to convert string numeric literal toNumber
- one of the operands is
Boolean
, the Boolean operand is converted to 1 or 0 Object
vsNumber
/String
: Object operand is converted to primitive value(if, and only if its comparand is a primitive), a String or a Number, using the valueOf and toString methods
13. what is the difference between var x = 1
and x = 1
? Answer in as much or as little detail as you feel comfortable
var x = 1
is explicit declaration while x = 1
is implicit declaration. It leads to
- Scope inheritance in case 2:
- if scope chain from execution context doen't declare(both explicit and implicit) variable x,
x
will declare and initialize on global withconfigurable = true
. - Otherwise
x
will reuse that declaration(in parent scope) and set value with1
.
- if scope chain from execution context doen't declare(both explicit and implicit) variable x,
- In case 1:
var x
will be declare inexecution context
with optionconfigurable = false
and also be hoisted.
- Creation Stage: create the
scope chain
, createvariable object
and determine the value ofthis
. Createvariable object
will lead:- Create
arguments object
, check the context for parameters, initialize the name and value and create a reference copy. - Scan the context for function declarations:
- For each function found, create a property in
variable object
which has a reference pointer to the function in memory. - If function name exists, the reference pointer value will be overwritten.
- For each function found, create a property in
- Scan the context for variable declarations:
- For each one found, create a property in
variable object
and initialize the value asundefined
. - If variable name already exists in the
variable object
, do nothing and continue scanning.
- For each one found, create a property in
- Create
- Activation / Code Execution Stage: Assign values, references to functions and execute code.
executionContextObj = {
scopeChain: { /* variableObject + all parent execution context's variableObject */ },
variableObject: { /* function arguments / parameters, inner variable and function declarations */ },
this: {}
}
14. Difference between Classical Inheritance and Prototypal Inheritance
Classical Inheritance | Prototypal Inheritance |
---|---|
Classes typically define the structure of objects | The objects themeselves define their own structure and this one can be inherited and modified by other object at the runtime |
It is static, means that after defining you can't modify its behavior at runtime | It is dynamic, you can add new properties to prototypes after they are created, this allows you to add new methods to a prototype which will be automatically made available to all the objects which delegate to that prototype |
It's quite complicate, containing many aspect like abstraction, encapsulation, inheritance and polymorphism therefore it provides some stuffs for you to easily achive OOP, also looks more naturally | It's simple, most of OOP aspect you need to implement to achive that |
Tight coupling, the gorilla / banna problem |
15. What is difference between private variable, public variable and static variable? How we achieve this in JS?
Public variable is visible in both current scope and other scopes through accessing.
Static variable belong to class intead of an instance.
function Variable() {
var private = 1;
this.public = 2;
}
Variable.static = 3;
16. How to achieve inheritance? Compare ways of inheritance. What are the downsides?
- Pseudo-classical pattern, assigning prototype to prototype.
- Function pattern, declaring attribute in side object and inherit through call it again.
- Prototypal pattern, using
Object.create
.
In pseudo-classical pattern, parent and child share same prototype. It leads drawback that when we modify child it will also change in parent.
In function pattern, they don't use prototype concept and is very strict to follow and everytime we create new object javascript engine need to recompile everything.
In prototypal pattern, it cames as standard of EMACScript 5 therefore it leads to browser compability.
17. Why extending array is bad idea?
Array.prototype
because it's much easier and more efficient
Array.prototype.last = function() {
return this[this.length - 1];
};
[1, 2, 3].last(); // 3
Array.prototype
comes with the price; that price is change of collisions. When scripts coexists with other scripts in an application, it's important for those scripts not to conflict with each other. Extending Array.prototype
while tempting and seemingly useful, unfortunately isn't very safe in a diverse environment. Different scripts can end up define same-named methods but with different behavior. Such behavior often lead to inconsitent behavior and hard-to-track errors.Collisions can happen not only with user-defined code but also with proprietary methods implements by environment itseft(e.g
Array.prototype.indexOf
) or from future standards
18. Explain how to prototypal inheritance works?
19. Explain function hoisting?
var
and function declaration
function hositing() {
// code here
var x = 1;
// code here
function action() {};
}
20. Explain event delegation (what's the difference between on and bind is in jQuery)?
event.target
There're two main benefits:
- Memory footprint which used by event listeners goes down.
- Also work for new element which is created dynamically.
- Most of event do bubble but not all of them.
- Delegation puts extra load on the browser because the handler runs when an event happens anywhere inside the container
21. What are promises?
- Promises: describe an object that acts as a proxy for a result that is normally not finished.
- Callback: is simply a function you pass into another function so that function can call it at a later time.
then
:
A promise is defined as an object that has a function as the value for the property then:
then(fulfilledHandler, errorHandler, progressHandler)
Adds afulfilledHandler
,errorHandler
, andprogressHandler
to be called for completion of a promise. ThefulfilledHandler
is called when the promise is fulfilled. TheerrorHandler
is called when a promise fails. TheprogressHandler
is called for progress events. All arguments are optional and non-function values are ignored. TheprogressHandler
is not only an optional argument, but progress events are purely optional. Promise implementors are not required to ever call aprogressHandler
(theprogressHandler
may be ignored), this parameter exists so that implementors may call it if they have progress events to report.
This function should return a new promise that is fulfilled when the givenfulfilledHandler
orerrorHandler
callback is finished. This allows promise operations to be chained together. The value returned from the callback handler is the fulfillment value for the returned promise. If the callback throws an error, the returned promise will be moved to failed state.
22. what are some things you can do to improve page loading speed?
- Should dynamic load javascript and css to prevent blocking render in DOM.
- Minimize the number of http requests to server as little as possible
- Combine multiple style sheets into one.
- Use css instead of images whenever possible.
- Reduce scripts and put them at the bottom of the page.
- Use image sprite.
- Reduce server response time(less then 200ms).
- Enable compression.
- Enable browser caching.
- Minify resources(html, css, javascript).
- Reduce the number of plugins you use on your site.
- Reduce redirects.
- Use a Content Delivery Network.
23. What is the significance of including 'use strict'
at the beginning of a JavaScript source file?
- security.
- to avoid what they consider to error-prone features.
- to get enhanced in error checking(means it will throw exceptions in situations that are not specified as errors in non-strict mode).
- other reasons of their choosing.
24. What's the different between function declaration and function expression
FunctionDeclaration :
function Identifier ( FormalParameterListopt ) { FunctionBody }
FunctionExpression :
function Identifieropt ( FormalParameterListopt ) { FunctionBody }
There're four ways to define function
- a function defined with the Function constructor assigned to the variable multiply
var multiply = new Function("x", "y", "return x * y;");
- a function declaration of a function named multiply
function multiply(x, y) {
return x + y;
}
- a function expression of an anonymous function assigned to the variable multiply
var multiply = function(x, y) {
return x + y;
}
- a function expression of a function named func_name assigned to the variable multiply
var multiply = function func_name(x, y) {
return x + y
}
- A function defined by a
Function
constructor does not inherit any scope other than the global scope - Functions defined by function expressions and declarations are parsed only once while those defined by the
Function
constructor are not.
Function constructor
string aren't parsed repeatlyMozilla function and function scope
25. How would you change the context of the function?
bind
, call
and apply
bind
: create function with new context by passing parameters.call
andapply
: call function with new context. They are different each other through the way they pass parameters to function
new
was called:
- It creates new object.
- It sets the constructor to object XXX.
- It sets up object to delegate to XXX.prototype.
- It call XXX() in the context of new object.
26. About typeof
and instanceOf
operators, constructor
property?
✍ All examples below are only in non-strict mode. strict mode is quite difference.
String.prototype.returnMe= function() {
return this;
}
var a = "abc";
var b = a.returnMe();
a; //"abc"
typeof a; //"string" (still a primitive)
b; //"abc"
typeof b; //"object"
a === b; // false
This is what realy happen
var primitive = "september";
primitive.vowels = 3;
//new object created to set property
(new String("september")).vowels = 3;
primitive.vowels;
//another new object created to retrieve property
(new String("september")).vowels; //undefined
typeof
: return built-in type, it has some issues in cases of null returnobject
, any object type other function returnobject
. Normally, people will define their own function instead oftypeof
usingObject.prototype.toString.call()
to get internal [[Class]].
var toType = function(obj) {
return ({}).toString.call(obj).match(/\s([a-zA-Z]+)/)[1].toLowerCase()
}
instanceOf
: check whether anything from left-hand object's prototype chain is the same object as what's reference by prototype property of right-hand object. The problem arise when it comes to scripting in multi-frame DOM environments instanceOf harmful
27. List every way the browser (using HTML, JS or CSS) can communicate with the server?
- Ajax
- Tag: Iframe, Embed, Object, Video, Audio, Image.
- Script tag injection
- Websocket
28. Using object as key?
string
, therefore if object is used as key, object will be converted to string
type via toString
method
✍ There're the definition I copy from Internet.
29. What's closure?
- Lexical scoping: is statically defined by the function's physical placement within the written source code.
function outter() {
var x = 1;
function inner() {
console.log(1)
}
}
- Dynamic scoping:
void dynamic() {
console.log(x)
}
void main() {
var x = 1;
dynamic();
}
.30 Callstack?
console.log('Hello');
setTimeout(function() { console.log('Hi'); });
for(var i = 0 ; i <= 10000 ; ++i) console.log('Instance ' + i);

Callback function only calls when current stack which contains this function is empty. In this case, it is called with this order
Hello
Intance 1
...
Instance 10000
Hi